一、专业术语解释
1、spring:是分层的Java SE/EE应用full - stack轻量级开源框架,以IoC(控制反转)和AOP(面向切面编程)为内核,提供展现层spring MVC 和 spring JDBC等众多企业级应用技术。
2、mybatis:是一个持久层框架。原始连接数据库是通过JDBC的API来操作的,过程繁琐,而mybatis是对JDBC的封装。
二、spring整合mybatis完整步骤
1、准备工作(新建项目及新建包)
1.1、
1.2、
1.3、
1.4、
1.5、
1.6、删除src包下,暂时不需要的包,同时在src/main包下新建java包、resource包以及在src包下新建test包和resource包。新建后的原始maven文件
1.7、删除掉main下的webapp包,接下来就是在main下新建几个包
步骤:file——project structure
1.8、 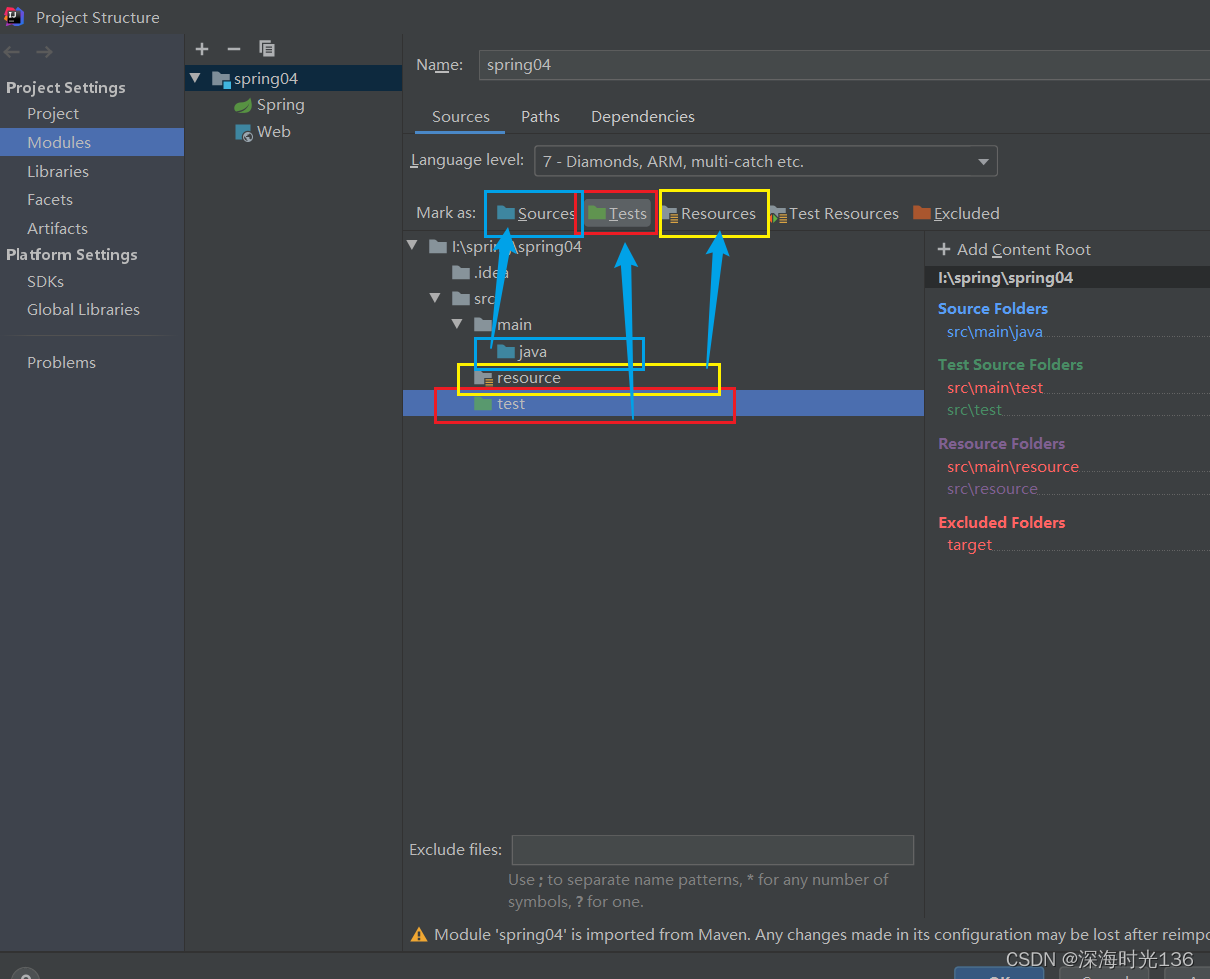
1.9、 再在java包下新建dao包、servcie包、entity包、config包,在resource包下新建一个文件jdbc.properties
dao包:数据层,在这里主要写操作数据库的接口
service包:逻辑控制层,主要处理一些逻辑,目前还用不上逻辑处理,主要是中转数据层
entity层:实体层,这一层主要存放数据信息,这里的数据和数据库信息保持一致
config包:这一层主要数存放spring的配置类、jdbc配置类和mybatis类
jdbc.properties:这个文件主要存放连接数据库的一些信息
1.10、准备工作的最后一步就是导入需要的架包了,spring整合mybatis所需架包如下
在pom.xml导入架包哈!导完架包后记得刷新一下maven
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<!-- spring架包-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.10.RELEASE</version>
</dependency>
<!-- 数据源-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.16</version>
</dependency>
<!-- mybatis包-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.4.5</version>
</dependency>
<!-- mybatis-spring-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.0</version>
</dependency>
<!-- mysql-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.49</version>
</dependency>
<!-- spring-jdbc-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.10.RELEASE</version>
</dependency>
<!-- 记录日志-->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
2、准备工作已经完成,现在就是整个项目的开始了
2.1、SpringConfig.java
package config;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Import;
import org.springframework.context.annotation.PropertySource;
//这个程序到时候由主程序创建一个对象引入
//开启纯注解模式
@Configuration
//既然已经开启了注解模式,那么主程序主要扫描那些包了
//扫描包
@ComponentScan({"dao","service"})
//jdbc.properties配置识别数据的一些信息,如何识别了
@PropertySource(value = "classpath:jdbc.properties",encoding = "utf-8")
//既然是spring整合mybatis,肯定有一个mybatis.java,所以新建mybatis类
//既然是连接数据库,连接数据库的信息写哪里了,所以再新建一个jdbcCOnfig类
//如何引入这个两个文件了
@Import({MybatisConfig.class,JdbcConfig.class})
public class SpringConfig {
}
2.2、jdbc.properties
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/user?characterEncoding=utf8&useSSL=false&serverTimezone=UTC&rewriteBatchedStatements=true
jdbc.username=root
jdbc.password=123456
2.3、jdbcConfig.java
package config;
import com.alibaba.druid.pool.DruidDataSource;
import org.springframework.beans.factory.annotation.Value;
public class JdbcConfig {
// 连接数据:数据库地址、数据源、数据库名、数据库密码
@Value("${jdbc.driver}")
private String driver;
@Value("${jdbc.url}")
private String url;
@Value("${jdbc.username}")
private String username;
@Value("${jdbc.password}")
private String password;
// 数据源
public DruidDataSource druidDataSource() {
DruidDataSource ds = new DruidDataSource();
ds.setDriverClassName(driver);
ds.setUrl(url);
ds.setUsername(username);
ds.setPassword(password);
return ds;
}
}
2.4、mybatis.java
package config;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.mapper.MapperScannerConfigurer;
import org.springframework.context.annotation.Bean;
import javax.sql.DataSource;
public class MybatisConfig {
@Bean
public SqlSessionFactoryBean sqlSessionFactoryBean(DataSource dataSource) {
SqlSessionFactoryBean ssfb = new SqlSessionFactoryBean();
// 扫描实体类
ssfb.setTypeAliasesPackage("entity");
// 加载数据源
ssfb.setDataSource(dataSource);
return ssfb;
}
// 接下来就是扫描dao层
public MapperScannerConfigurer mapperScannerConfigurer() {
MapperScannerConfigurer maper = new MapperScannerConfigurer();
maper.setBasePackage("dao");
return maper;
}
}
2.5、entity层 student类
package entity;
public class Student {
private int id;
private String name;
private String sex;
public Student(int id, String name, String sex) {
this.id = id;
this.name = name;
this.sex = sex;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", sex='" + sex + '\'' +
'}';
}
}
向数据库添加两条数据
2.6、dao层,主要通过接口中mysql语句来对数据库操作
package dao;
import entity.Student;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface StudentDao {
// 查询
@Select("SELECT * FROM student")
List<Student> queryAll();
}
2.7、 service层,分别新建接口和实现类
实现类:
package service.Impl;
import dao.StudentDao;
import entity.Student;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import service.StudentService;
import java.util.List;
@Service("StudentService")
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentDao studentDao;
public List<Student> queryAll() {
return studentDao.queryAll();
}
}
接口类
package service;
import entity.Student;
import java.util.List;
public interface StudentService {
List<Student> queryAll();
}
2.8、以上是一个spring整合mybatis的简单查询功能,先来测试一下,然后再做增删查改
import config.SpringConfig;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import service.StudentService;
public class StudentTest {
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringConfig.class);
StudentService studentServices = ctx.getBean(StudentService.class);
System.out.println(studentServices.queryAll());
}
}
3、增加数据:即向数据库中插入一条数据,写代码的顺序是,现在dao层写一个方法和mysql语句——再去service层的实现类调用dao和对实现类的方法做一个接口——再去测试类中新建main函数,调用service层接口,这就是整个项目的流程
3.1、在dao层写接口方法和mysql语句
package dao;
import entity.Student;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.Update;
import java.util.List;
public interface StudentDao {
// 查询
@Select("SELECT * FROM student")
List<Student> queryAll();
// 增加数据
@Insert("INSERT INTO student(id,name,sex) VALUE(#{id},#{name},#{sex})")
void insert(Student student);
// 按id值查询
@Select("SELECT * FROM student WHERE id = #{id}")
List<Student> findId(Integer id);
// 删除数据,根据id来删除数据
@Delete("DELETE FROM student WHERE id = #{id}")
void delete(Integer id);
// 更新数据
@Update("INSERT INTO student(id,name,sex) VALUE(#{id},#{name},#{sex})")
void update(Student student);
}
3.2、service层
实现类:
package service.Impl;
import dao.StudentDao;
import entity.Student;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import service.StudentService;
import java.util.List;
@Service("StudentService")
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentDao studentDao;
public List<Student> queryAll() {
return studentDao.queryAll();
}
public void insert(Student student) {
studentDao.insert(student);
}
public List<Student> findId(Integer id) {
return studentDao.findId(id);
}
public void delete(Integer id) {
studentDao.delete(id);
}
public void update(Student student) {
studentDao.insert(student);
}
}
接口
package service;
import entity.Student;
import java.util.List;
public interface StudentService {
List<Student> queryAll();
void insert(Student student);
List<Student> findId(Integer id);
void delete(Integer id);
void update(Student student);
}
3.3、测试
import config.SpringConfig;
import entity.Student;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import service.StudentService;
public class StudentTest {
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringConfig.class);
StudentService studentServices = ctx.getBean(StudentService.class);
System.out.println(studentServices.queryAll());
Student stu1 = new Student(202366,"chatGPT","Ai");
studentServices.insert(stu1);
System.out.println(studentServices.findId(202366));
studentServices.delete(202302);
Student stu2 = new Student(202399,"王五","男");
studentServices.update(stu2);
System.out.println(studentServices.queryAll());
}
}
3.4、增删改查
以上就是spring整合mybatis的全过程!!!
版权归原作者 深海时光136 所有, 如有侵权,请联系我们删除。