一、该图书管理系统涉及Java的知识点,如下:
- 数组的增删查
- 抽象类
- 接口
- 面向对象的封装、继承和多态
二、该图书管理系统包含的功能,如下:
- 图书管理系统的使用人群分为两种:①管理人员,②普通用户
- 具体实现:抽象类的继承
User类(父类):
public abstract class User {
protected String name;
protected IOperation[] iOperations;
public User(String name){
this.name=name;
}
public abstract int menu();
public void doWork(int choice, BooklList booklList){
iOperations[choice].work(booklList);
}
}
AdminUser类(管理员)
public class AdminUser extends User {
public AdminUser(String name){
super(name);
this.iOperations=new IOperation[] {
new ExitOperation(),
new FindOperation(),
new AddOperation(),
new DelOperation(),
new DisplayOperation()
};
}
public int menu(){
System.out.println("========管理员菜单========");
System.out.println("hello "+this.name +"欢迎使用图书管理系统");
System.out.println("1.查找图书");
System.out.println("2.新增图书");
System.out.println("3.删除图书");
System.out.println("4.显示图书");
System.out.println("0.退出系统");
System.out.println("==============================");
Scanner scanner=new Scanner(System.in);
int choice=scanner.nextInt();
return choice;
}
}
** NormalUser类(普通用户类)**
public class NormalUser extends User {
public NormalUser(String name){
super(name);
this.iOperations=new IOperation[]{
new ExitOperation(),
new FindOperation(),
new BorrowOperation(),
new ReturnOperation()
};
}
public int menu(){
System.out.println("========普通用户菜单========");
System.out.println("hello "+this.name +"欢迎使用图书管理系统");
System.out.println("1.查找图书");
System.out.println("2.借阅图书");
System.out.println("3.归还图书");
System.out.println("0.推出系统");
System.out.println("==============================");
Scanner scanner=new Scanner(System.in);
int choice=scanner.nextInt();
return choice;
}
}
注:需要把上述的三各类放在同一个包里
** 2. 设计一个Book类存放书的属性,设计一个BookList类存放书的位置**
具体实现:在Book类中给所有的私有属性提供了公共的接口,在BookList类中创建了一个名为books的数组并存放三本图书另外设计了一个BookList的成员名为UsedSize来表示在books数组中存放书的数量。
Book类
public class Book {
private String name;//书名
private String author;//书的作者
private int price;//书的价格
private String type;//书的类型
private boolean isBorrowed;//是否借出
public Book(String name, String author, int price, String type) {
this.name = name;
this.author = author;
this.price = price;
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public boolean isBorrowed() {
return isBorrowed;
}
public void setBorrowed(boolean borrowed) {
isBorrowed = borrowed;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", price=" + price +
", type='" + type + '\'' +
((isBorrowed==true)?" 已经被借出 ":" 未被借出 ")+
'}';
}
}
BookList类
public class BooklList {
private Book[] books=new Book[1024];
private int usedSize;
public BooklList(){
books[0]=new Book("数据库应用原理","张三",45,"计算机");
books[1]=new Book("红楼梦","曹雪芹",56,"小说");
books[2]=new Book("唐诗三百首","孙洙",35,"古诗词");
this.usedSize=3;
}
public int getUsedSize() {
return usedSize;
}
public void setUsedSize(int usedSize) {
this.usedSize = usedSize;
}
/**
* 获得pos位置的一本书
* @param pos
* @return
*/
public Book getPos(int pos){
if(pos>=0&&pos<books.length){
return this.books[pos];
}else
System.out.println("pos位置不合法");
return this.books[pos];
}
/**
* 添加pos下标的一本书
* @param pos
* @param book
*/
public void setBook(int pos,Book book){
this.books[pos]=book;
}
}
注: 需要把上述两个类放在当一个包里
3. 分别设计了七个类,每一个类实现一种功能;设计了一个接口把七个类串接起来
具体实现:AddOperation(增加操作)、BorrowOperation(借阅操作)、DelOperation(删除操作)、DisplayOperation(显示操作)、ExitOperation(退出系统)、FindOperation(查找操作)、ReturnOperation(归还操作)和接口IOperation
AddOperation(增加操作)
public class AddOperation implements IOperation{
public void work(BooklList booklList){
System.out.println("新增图书!");
System.out.println("请输入图书的名字:");
String name=scanner.nextLine();
System.out.println("请输入图书的作者");
String author=scanner.nextLine();
System.out.println("请输入图书类型");
String type=scanner.nextLine();
System.out.println("请输入图书价格");
int price=scanner.nextInt();
scanner.nextLine();
Book book=new Book(name,author,price,type);
int size=booklList.getUsedSize();
booklList.setBook(size,book);
booklList.setUsedSize(size+1);
System.out.println("新增图书成功!");
}
}
BorrowOperation(借阅操作)
public class BorrowOperation implements IOperation {
public void work(BooklList booklList) {
System.out.println("借阅图书!");
System.out.println("请输入你想借阅的图书名字");
String name = scanner.nextLine();
int size = booklList.getUsedSize();
for (int i = 0; i < size; i++) {
Book book = booklList.getPos(i);
if (name.equals(book.getName())) {
book.setBorrowed(true);
System.out.println("借阅成功!!!");
System.out.println("借阅图书信息如下:" + book);
return;
}
}
System.out.println("没有找到你要借阅的图书!!!");
}
}
DelOperation(删除操作)
public class DelOperation implements IOperation {
public void work(BooklList booklList) {
System.out.println("删除图书!");
System.out.println("请输入你要删除的图书的名字");
String name = scanner.nextLine();
int currentSize = booklList.getUsedSize();
int index = 0;
int i = 0;
for (; i < currentSize; i++) {
Book book=booklList.getPos(i);
if (book.getName().equals(name)){
index=i;
break;
}
}
if (i>=currentSize){
System.out.println("没有找打你要删除的图书!!!");
return;
}
for (int j = index; j <currentSize-1 ; j++) {
Book book=booklList.getPos(j+1);
booklList.setBook(j,book);
}
booklList.setBook(currentSize-1,null);
booklList.setUsedSize(currentSize-1);
System.out.println("删除成功!!!");
}
}
DisplayOperation(显示操作)
public class DisplayOperation implements IOperation{
public void work(BooklList booklList){
System.out.println("打印图书!");
int size=booklList.getUsedSize();
for (int i = 0; i <size ; i++) {
Book book=booklList.getPos(i);
System.out.println(book);
}
}
}
ExitOperation(退出系统)
public class ExitOperation implements IOperation{
public void work(BooklList booklList){
System.out.println("退出系统!");
System.exit(0);
}
}
FindOperation(查找操作)
public class FindOperation implements IOperation{
public void work(BooklList booklList){
System.out.println("查找图书!");
System.out.println("请输入你要查找的图书名字");
String name=scanner.nextLine();
int size=booklList.getUsedSize();
for (int i = 0; i <size ; i++) {
Book book=booklList.getPos(i);
if (name.equals(book.getName())) {
System.out.println("找到了这本书,信息如下:");
System.out.println(book);
return;
}
}
System.out.println("没有找到你需要借阅的图书!!!");
}
}
ReturnOperation(归还操作)
public class ReturnOperation implements IOperation {
public void work(BooklList booklList) {
System.out.println("归还图书!");
System.out.println("请输入你要图书的名字");
String name = scanner.nextLine();
int size = booklList.getUsedSize();
for (int i = 0; i < size; i++) {
Book book = booklList.getPos(i);
if (name.equals(book.getName())) {
book.setBorrowed(false);
System.out.println("归还成功!!!");
System.out.println("归还图书信息如下:"+book);
return;
}
}
System.out.println("没有你要归还的图书!!!");
}
}
接口IOperation
public interface IOperation {
void work(BooklList booklList);
Scanner scanner=new Scanner(System.in);
}
注:上述的七个类和一个接口需要放在同一个包里
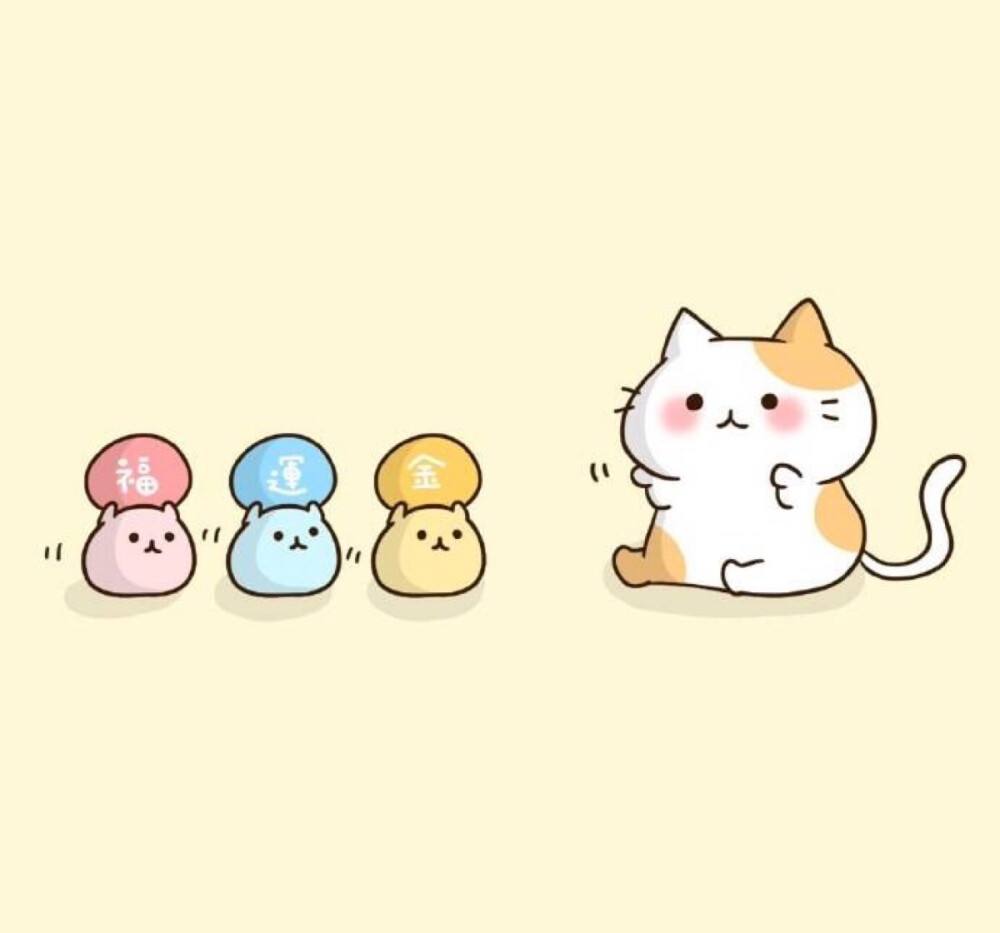 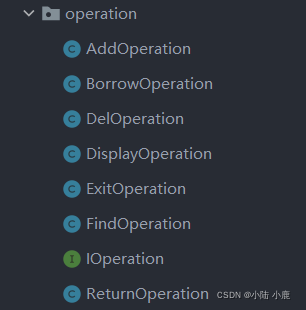
3. 主类Main,包含一个静态方法Login
Main类
public class Main {
public static User login(){
System.out.println("请输入你的姓名");
Scanner scanner=new Scanner(System.in);
String name=scanner.nextLine();
System.out.println("请输入你的身份:1——》管理员,2——》普通用户");
int choice=scanner.nextInt();
if(choice==1){
return new AdminUser(name);
}else {
return new NormalUser(name);
}
}
public static void main(String[] args) {
BooklList booklList=new BooklList();
User user=login();//向上转型
while (true) {
int choice = user.menu();//动态绑定,根据choice选择合适的操作
user.doWork(choice, booklList);
}
}
}
**4.结果图演示 **
管理员功能演示
** 普通用户功能演示**
版权归原作者 小陆 小鹿 所有, 如有侵权,请联系我们删除。