目录
1、项目介绍
Com.Gitusme.Net.Extensiones.Core 是一个 C#.Net 扩展库。当前最新 1.0.7 版本,使用方便快捷,能够减少代码量,提高编程效率。基于 .Net Standard 2.0 开发,支持 windows、linux、IOS 等多平台。
2、集成方式
方法一:项目中通过Nuget包管理器安装导入
选择项目,右键菜单,打开 NuGet 程序包 管理,搜索 ”Com.Gitusme.Net.Extensiones.Core“ 关键字,安装最新版本。
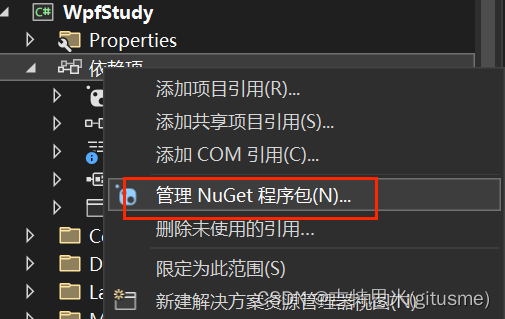
方法二:手动从Nuget官网下载,下载地址:
https://www.nuget.org/packages/Com.Gitusme.Net.Extensiones.Core
3、代码中导入命名空间
using Com.Gitusme.Net.Extensions.Core;
4、版本变更说明
1.0.7 版本- 修复Sokcet套接字重大BUG,增强可靠性;
1.0.6 版本- 新增Sokcet套接字扩展。简化Socket操作,支持自定义命令封装,使用方便快捷,让您聚焦业务实现,而不必关心底层逻辑,提高开发效率。详情请参考演示示例章节。- 日志功能增强。新版本支持日志的相关参数配置,默认文件路径为程序集所在目录,文件名: Com.Gitusme.Net.Extensiones.Core.Settings.xml
1.0.5 版本- 新增日志记录功能,支持 DEBUG,INFO,WARN,ERROR 级别,默认日志输出路径为程序集所在同级Log文件夹。使用方法简单,在任意class中,通过 this 打印日志。详情请参考演示示例章节。
1.0.4 版本- 新增 String,Object 类扩展,提供方便快捷的类型转换。详情请参考演示示例章节。
5、演示示例
示例 1:使用string, object扩展进行类型转换
** // Example 1: 判断string是否为null**
string str = null;
if (str.IsNullOrEmpty())
{
System.Console.WriteLine("Example 1 输出结果:" + "null");
}
** // Example 2: 判断string是否匹配正则**
string hello = "Hello, gitusme";
var isMatch = hello.IsMatch(@"Hello, \w+");
System.Console.WriteLine("Example 2 输出结果:" + isMatch);
**// Example 3: 将string转换为Json实体对象**
string json = "{\"Id\":0,\"Name\":\"Json Object\"}";
var jsonObj = json.ToJsonObject<MyJsonObject>();
System.Console.WriteLine("Example 3 输出结果:" + jsonObj.Name);
** // Example 4: 将string转换为Xml实体对象**
string xml = "<?xml version=\"1.0\" encoding=\"utf-8\"?>" +
"<root xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\" xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" id=\"0\">" +
"<name>Xml Object</name>" +
"</root>";
var xmlObj = xml.ToXmlObject<MyXmlObject>();
System.Console.WriteLine("Example 4 输出结果:" + xmlObj.Name);
**// Example 5: 将string转换为DateTime**
string date = "2023/10/22 21:32:00";
var dateTime = date.ToDateTime();
System.Console.WriteLine("Example 5 输出结果:" + dateTime.ToString());
** // Example 6: 将string转换为字符数组**
string gitusme = "gitusme";
var array = gitusme.ToCharArray();
System.Console.WriteLine("Example 6 输出结果:" + array);
** // Example 7: 将string转换为int32**
string intStr = "100";
var intValue = intStr.ToInt32() + 10;
System.Console.WriteLine("Example 7 输出结果:" + intValue);
**// Example 8: 将string转换为decimal**
string decimalStr = "3.141592653589793238462643383279502884197";
var decimalValue = decimalStr.ToDecimal();
System.Console.WriteLine("Example 8 输出结果:" + decimalValue);
**// Example 9: 将string转换为uri**
string uriStr = "https://github.com/gitusme/Com.Gitusme.Net.Extensiones";
var uriValue = uriStr.ToUri();
System.Console.WriteLine("Example 9 输出结果:" + uriValue.Host);
**// Example 10: 将string转换为color**
string colorStr = "#aabbcc";
var colorValue = colorStr.ToColor();
System.Console.WriteLine("Example 10 输出结果:" + colorValue);
运行结果:
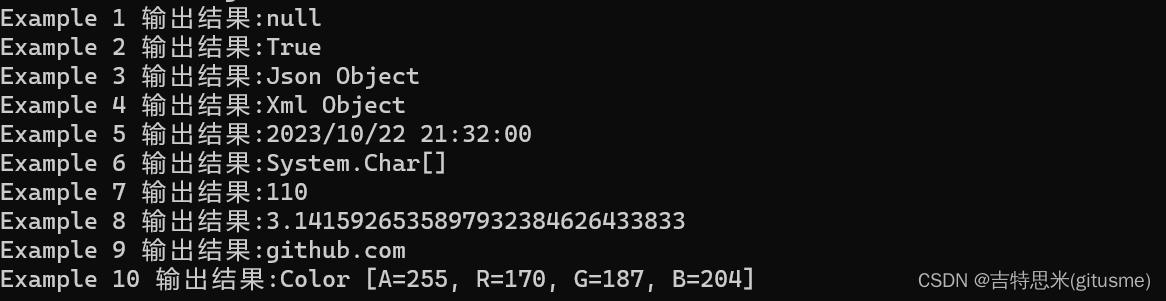
** // Example 1: 判断对象是否为null**
MyObject obj = null;
if (obj.IsNull())
{
System.Console.WriteLine("Example 1 输出结果:" + "null");
}
**// Example 2: 对象为null的时候,返回传入的默认值**
var def = obj.OrDefault(new MyObject());
System.Console.WriteLine("Example 2 输出结果:" + def);
**// Example 3: 如果对象不为null,则执行传入的Action行为**
def.IfPresent((it) =>
{
System.Console.WriteLine("Example 3 输出结果:" + it.Name);
});
**// Example 4: 将Xml对象转换为Xml文本**
var xmlObj = new MyXmlObject();
string xml = xmlObj.ToXml();
System.Console.WriteLine("Example 4 输出结果:" + xml);
**// Example 5: 将Json对象转换为Json文本**
var jsonObj = new MyJsonObject();
string json = jsonObj.ToJson();
System.Console.WriteLine("Example 5 输出结果:" + json);
运行结果:

示例 2:使用this指针在任意类中打印日志
class MyServer
{
public void Start(string arg1, string arg2)
{
this.Logi("MyServer", "Start:args={0},{1}", arg1, arg2);
}
public void Stop()
{
this.Logi("Stop.....");
}
}
日志输出:
2023-11-04 18:25:16.158 [INFO] [00001] MyServer: Start:args=arg1,arg2
2023-11-04 18:25:16.261 [INFO] [00001] MyServer: Stop.....
示例 3:创建Socket服务端与客户端通信
服务端代码:
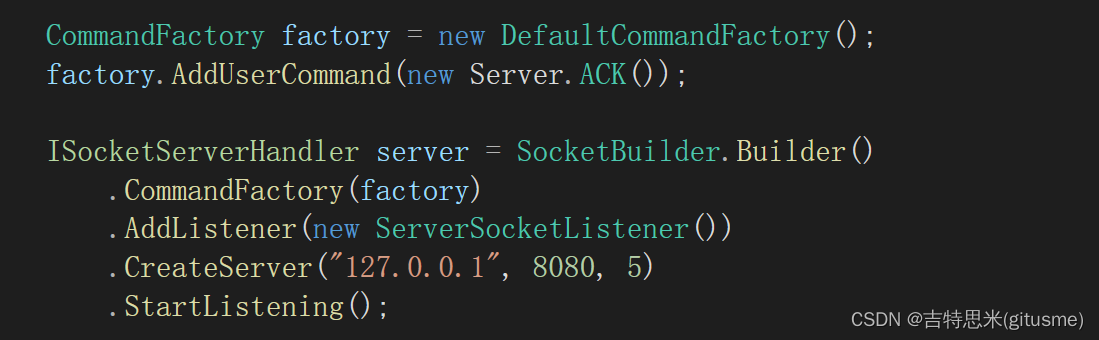
客户端代码:
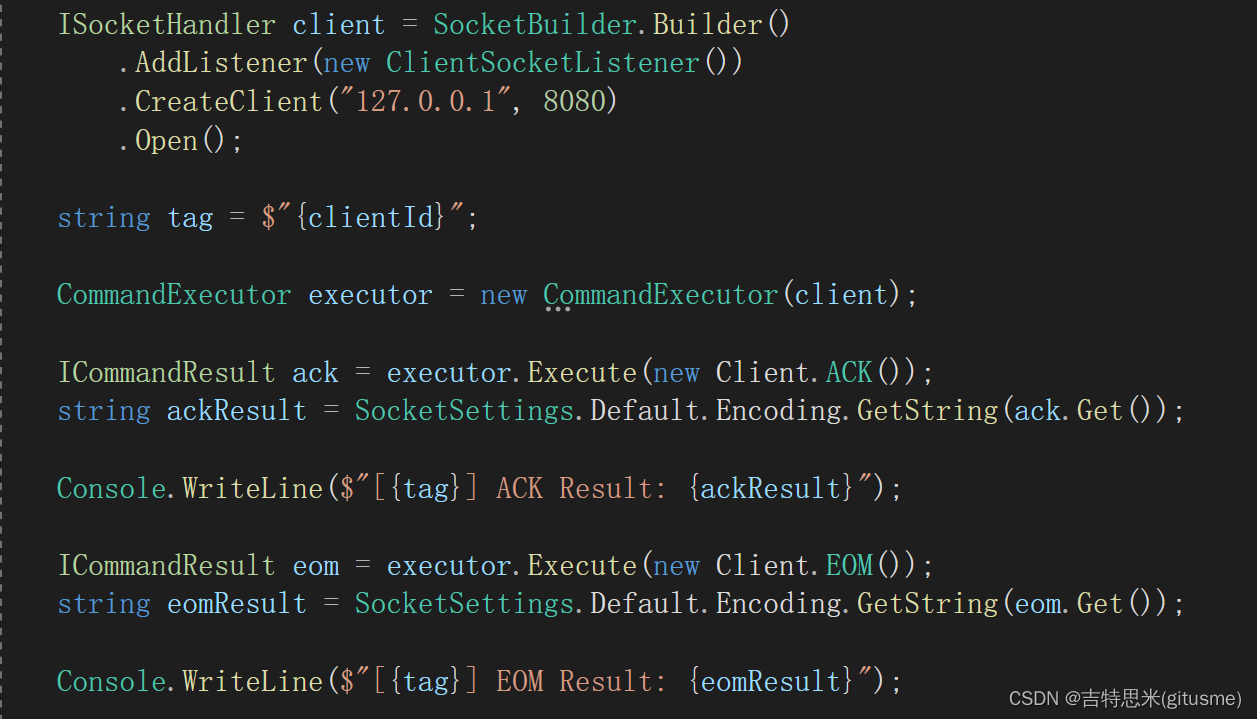
运行结果:

如上示例,演示服务器和客户端的创建和请求应答交互,如需新增命令,可以从 AbstractCommand 派生实现自己的命令,通过CommandFactory来管理命令集。提供ServerSocketListener 和 ClientSocketListener 接口,分别监听服务器和客户端的生命周期。服务器使用 ServerSocketListener.OnAccepted(commandFilter, ISocketHander acceptHandler) 监听新的客户端连接,通过 acceptHandler 来操作客户端的消息交互。
可靠性验证:完美执行10000次Socket创建和收发数据,未出现异常。
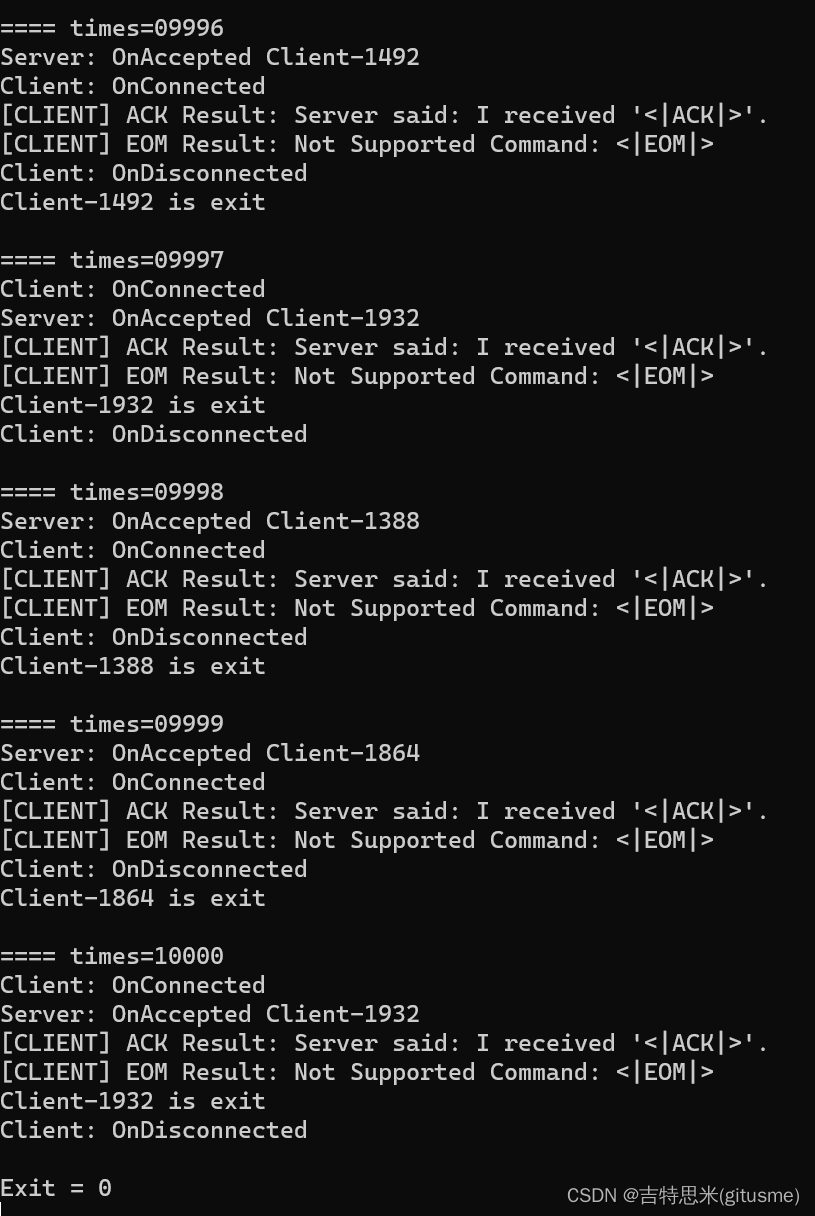
最后,感谢您能抽出宝贵的时间认真学习完本篇,实属不易。如果您有好的想法或建议,欢迎点赞加关注,收藏交流和探讨。
版权归原作者 吉特思米(gitusme) 所有, 如有侵权,请联系我们删除。